Breaking Down the CSS Syntax
π‘
Essential Question: Essential Question: How do you “select” an HTML element in CSS?
OK, so we know what “CSS” means, but how do you write CSS code? It’s a good idea to familiarize ourselves with the way CSS is written before we get to actually writing it. That’s exactly what we’ll learn in this lesson.</p
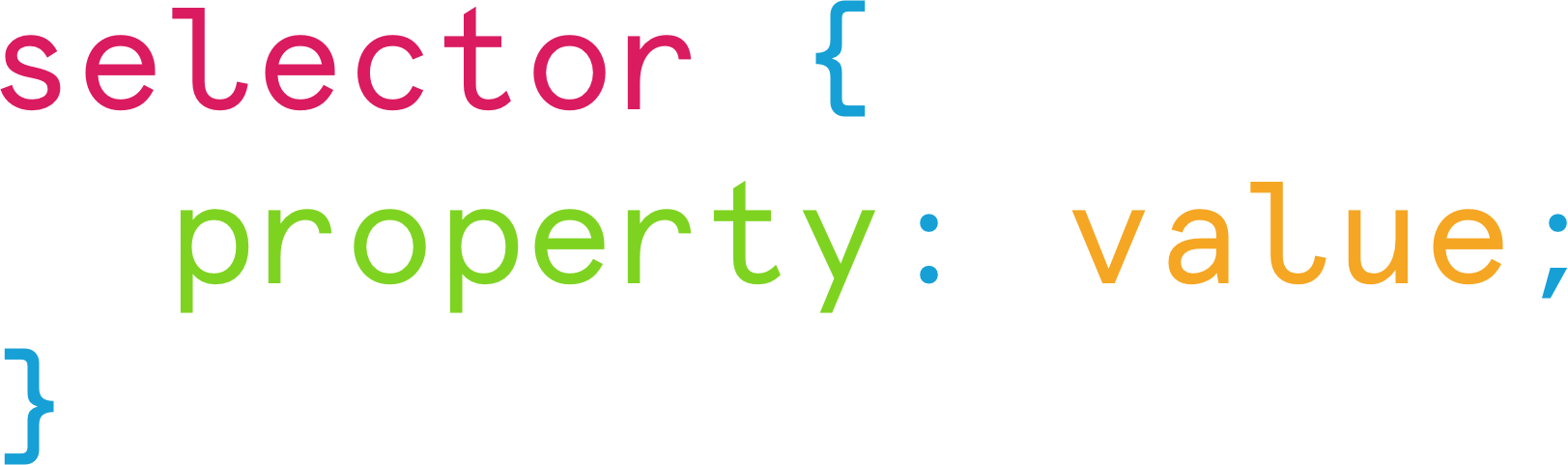
Selectors
Here’s the basic idea of CSS: you select something, then apply styles to it. We call the things that we style selectors in CSS. If you ever see the word “selector” in this course, what we’re referring to is the thing that we’re styling.
An HTML element can be a selector. Say we want to style a <header>
element in the HTML:
header {
property: value;
}
That’s it! All it takes to style a head is to write the name of the HTML element β nothing else. The thing about styling an element like this, however, is that now CSS is going to style any and all <header>
elements. That’s because all we’re telling CSS is to select anything that is a header and apply certain styles to it.
If we want to get more specific, then we have to look at CSS classes and IDs.
CSS Classes & IDs
A more specific way to select elements in CSS is by using classes and IDs. Imagine for a moment that we have the following HTML:
<article>
<!-- etc. -->
</article>
<article>
<!-- etc. -->
</article>
We know that we can use <article>
as the selector to style both articles at the same time:
article {
color: #000;
}
But let’s say that we want the background color of one article to be white (#fff
) with black (#000
) text and the other to be the reverse of that with a white background and black text.
Using IDs
We can differentiate between the two by using either classes or IDs. Let’s update the HTML so each article has a unique ID name:
<article id="articleDark">
<!-- etc. -->
</article>
<article id="articleLight">
<!-- etc. -->
</article>
Now we can style each article separately using the IDs as selectors, using a hash (#
) sign before the ID name:
#articleDark {
background-color: #000;
color: #fff;
}
#articleLight {
background-color: #fff;
color: #000;
}
Nice! Now we have two differently-styled articles!
Using classes
Classes in CSS look almost exactly like IDs, but use a period (.
) instead of a hash. So, given the following HTML:
<article class="article-dark">
<!-- etc. -->
</article>
We can select that article using its classname like this:
.article-dark {
color: #000;
}
Naming IDs and classes
If you’re wondering how I came up with those specific ID names, they are totally arbitrary. You can name both IDs and classes however you want. That said, I find it’s a good idea to find a consistent format because naming things is hard. I personally write IDs in a camelCase format, and classes using a BEM format.
Which one should you use?
Using IDs for selectors is great! But in most cases, you’ll see developers use classes instead. That’s because IDs are way higher up in the CSS Cascade than classes, which makes them harder to override with other CSS. If we have an element with both an ID and a class in the HTML, like this:
<article id="articleDark" class="article-dark">
<!-- etc. -->
</article>
Now let’s try to override the black text in the ID with dark gray text in a class selector:
/* ID */
#articleDark {
color: #000; /* This color wins */
}
/* Class */
.article-dark {
color: #333;
}
That’s right: the IDs color wins, even though the class is lower in the Cascade. Using classes is a much more flexible way to style elements. I’d suggest limiting your use of IDs to super special cases where you do not a certain style to be easily overridden. In fact, you will see classes used more often in modern web development.
CSS Properties and Values
Now that we know how to select elements with CSS, we need to apply styles to them. And the way we apply styles to HTML elements in CSS is with CSS properties and values. The two always come together as a pair and are often referred to as property:value keys.
A CSS property is the type of style that’s being added. In the example above, we wanted to style anΒ <article>
element’s text color. The property for text color isΒ color
.
A CSS value is how we tell CSS how we want to style a property. Again, in the example above, we wanted the <article>
element’s text color or color
to be black. That means #000
is the value of the color property.
.article-dark {
/* Property:Value combination */
color: #000;
}
How many properties and values are there in CSS? Lots of them! It would take up a book’s worth of space to name and define them all, so I highly suggest bookmarking Mozilla Developer Network’s reference of CSS properties for an exhaustive list of everything you can refer to any time you need it β and we all do at some point, even those of us who have been writing CSS for years!
There’s also a shorter list of the most commonly used CSS properties if you want something that’s a little easier to digest. We’ll be covering specific properties throughout this course, so don’t feel any pressure to start memorizing stuff right away.
Bringing it All Together!
We have selectors, properties, and values in CSS. Taken together, we call this group of code a CSS ruleset. I like that name because what we’re writing is literally a set of rules we’re telling the browser to use to style elements on the page.
/* A complete ruleset! */
.my-element {
background-color: #000;
border: 1px solid #f8a100;
border-radius: 16px;
color: #fff;
padding: 1.5rem;
}
Don’t worry, there’s no need to know what all those properties do just yet. We’re still figuring out how to write CSS!
βΆοΈ Watch: Classes & IDs
In the following video, Kevin Powell compares the differences between the two most common types of CSS selectors and when to use them. Pay special attention to the difference between IDs and classes, as they are often confused and lead to issues managing the CSS Cascade.
Check Your Understanding
Please answer the following ungraded questions to help you determine whether you are ready to proceed to the next lesson in the module.
(1) What are three types of CSS selectors?
Show the Answer
- Element selectors (e.g.
header
selects a<header>
element) - ID selector (e.g.
#cardTitle
selects an element that matches<div id="cardTitle">
) - Class selector (e.g.
#cardTitle
selects an element that matches<div class="card-title">
)
(2) Why is it generally a good idea to use a class selector instead of an ID selector?
Show the Answer
IDs carry greater weight than classes do in the CSS Cascade. That means an ID may apply a style higher up in the Cascade tha en a class that is lowerof in the Cascade, despite coming after the ID. This creates inconsistent behavior in the Cascade and makes it more difficult to override an ID style with a class selector.
(3) What is a property:value pair?
Show the Answer
A property is the type of style we want to apply to a certain selector. For example, if we want to change the text color of an element in HTML, then we would select the element and declare the color
property.
A value is the configuration of the property. For example, to set the color
property to a specific color, we would declare a color value (e.g. color: black
).
A property is declared before the value, and they are separated by a colon, resulting in a property:value pair. A collection of property:value pairs inside of a selector is called a CSS ruleset.